Setup reCaptcha in Angular
How to setup reCaptcha in Angular using ng-reCaptcha
Setting up reCaptcha
becomes necessary to provide good security to front end applications. In this article we are going to be setting up reCaptcha
in Angular.
Here are going to be using a library called called ng-recaptcha
. Setting up reCaptcha
in Angular is made simple using ng-recaptcha
library. Follow below steps to setup reCaptcha
.
-
Install
ng-recaptcha
library using commandnpm install ng-recaptcha --save
-
To setup
reCaptcha
we need to getsiteKey
from google reCaptcha site. You can it from here -
Fill the details. Provide your domain name.
For development purposes also provide domain name
localhost
along with you production domain. Once you push to production removelocalhost
.
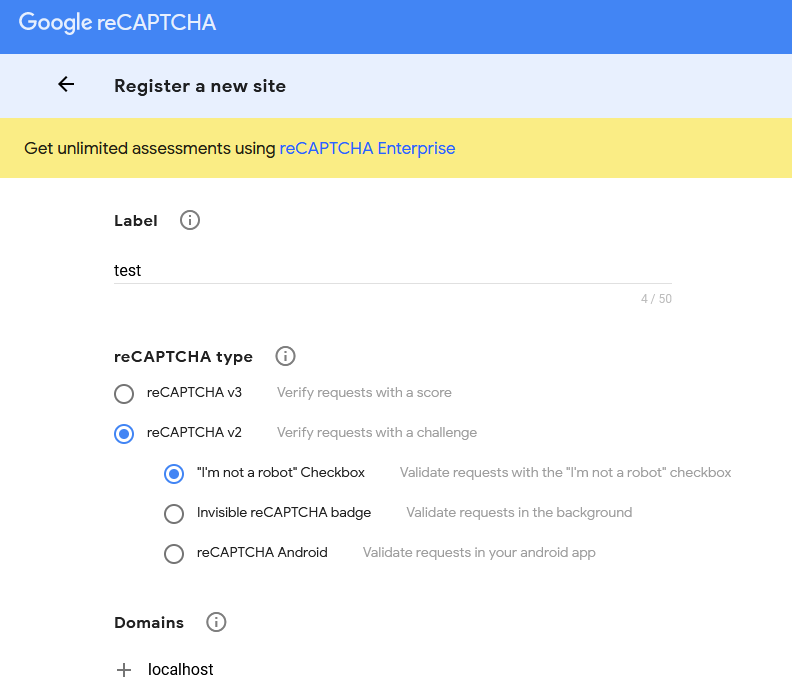
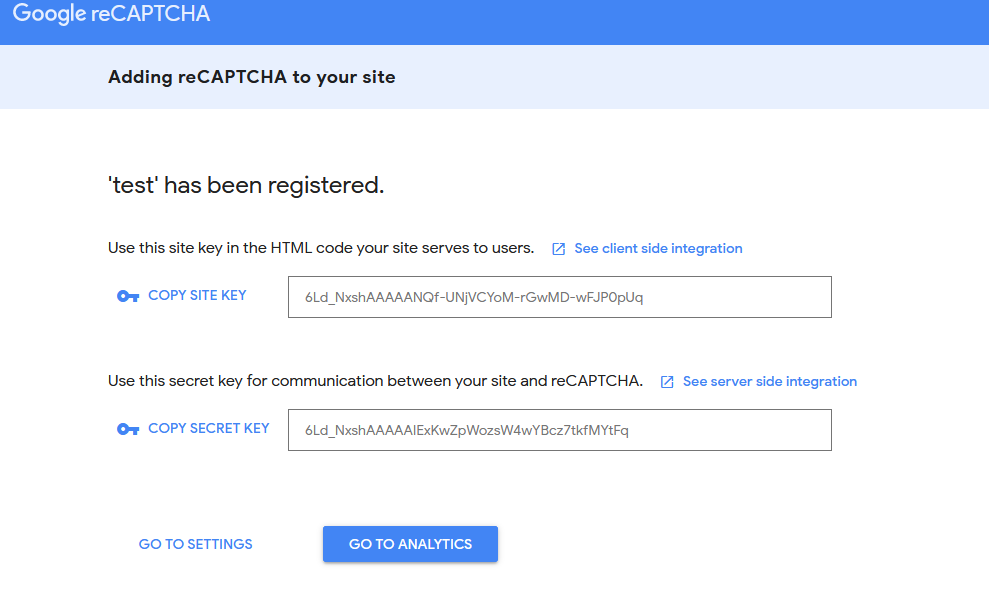
-
Once we obtain the
site key
, we need to add below tag in angular html file.<re-captcha (resolved)="reCaptchaResolved($event)" siteKey="{{siteKey}}"></re-captcha>
-
In your
NgModule
, addRecaptchaModule
under imports. -
If
siteKey
is correct it should display thereCaptcha
. -
If we want capture
reCaptcha
event, then we can use theresolved
event to attach a method. We can do further business logic here. In our example we defined it as(resolved)="reCaptchaResolved($event)"
.FYI , In our component ts file we have define
reCaptchaResolved
method.
Add reCaptcha
without ng-recaptcha
We don’t need use ng-recaptcha
to setup recaptcha
in Angular. ng-recaptcha
just makes it easier.
To implement reCaptcha
without using ng-recaptcha
we need to follow below mentioned steps,
-
Add below div in your angular html file,
<div class="g-recaptcha" data-sitekey="{{sitekey}}" data-callback="reCaptchaResolved" id="capcha_element"></div>
-
Add below code in the ts file
declare var grecaptcha: any; grecaptcha.render('capcha_element',{'sitekey': this.siteKey});
-
Now finally in your index.html add below script tag,
<script src='https://www.google.com/recaptcha/api.js'></script>
-
After this
recaptcha
should be visible
Change language in recaptcha
-
Google
reCaptcha
will detect browser language and change the language according to the browser setting. -
But if you want to change language explicitly, you can do it in
index.html
. Addhl
parameter in the scriptsrc
tag, for example if we want to change language tofrench
then you need edit script tag to as shown below.<script src='https://www.google.com/recaptcha/api.js?hl=fr'></script>
Following the above mentioned steps we can easily setup reCaptcha
in an Angular application.
For more articles on Angular check here.